What is Algorithm?
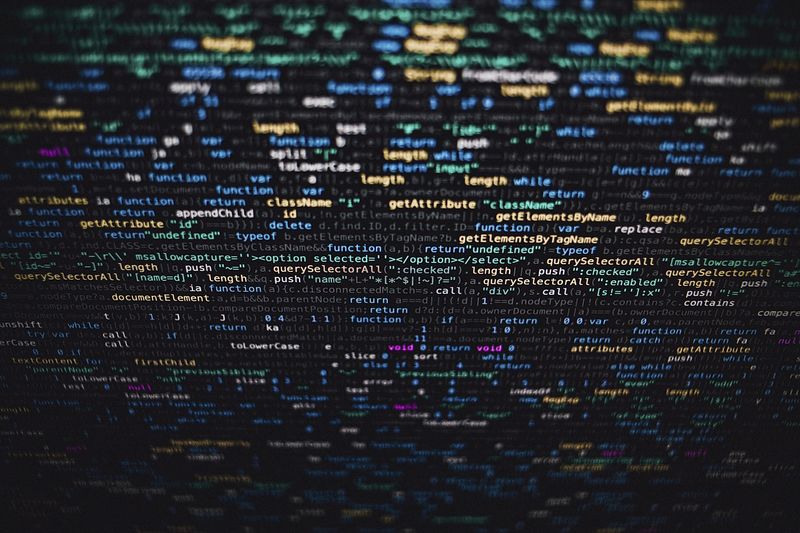
An algorithm is a step-by-step procedure or set of rules for solving a problem or performing a task. In computer science and mathematics, algorithms are fundamental for designing software and systems. They provide a clear and structured approach to process data, make decisions, and produce results.
Key Features of Algorithms:
1. Defined Instructions
- Clear Steps: An algorithm consists of a sequence of well-defined, unambiguous instructions that lead to a specific outcome.
- Order of Execution: The instructions must be executed in a particular order to achieve the desired result.
2. Input and Output
- Input: An algorithm takes input data or parameters that it processes.
- Output: It produces output based on the input data and the steps defined.
3. Finiteness
- Termination: An algorithm must terminate after a finite number of steps. It should not run indefinitely.
4. Effectiveness
- Practicality: The instructions must be simple enough to be executed, either by a computer or manually, and must achieve the desired outcome.
Types of Algorithms:
1. Sorting Algorithms
- Purpose: Arrange data in a particular order (e.g., ascending or descending).
- Examples: Bubble Sort, Merge Sort, Quick Sort.
2. Searching Algorithms
- Purpose: Find specific data within a data structure.
- Examples: Binary Search, Linear Search.
3. Graph Algorithms
- Purpose: Solve problems related to graphs, such as finding the shortest path or traversing nodes.
- Examples: Dijkstra’s Algorithm, Depth-First Search (DFS), Breadth-First Search (BFS).
4. Dynamic Programming Algorithms
- Purpose: Solve problems by breaking them into simpler subproblems and storing the results of these subproblems to avoid redundant computations.
- Examples: Fibonacci Sequence, Knapsack Problem.
5. Greedy Algorithms
- Purpose: Make the locally optimal choice at each step with the hope of finding a global optimum.
- Examples: Kruskal’s Algorithm for Minimum Spanning Tree, Huffman Coding.
Algorithm Design Paradigms:
1. Divide and Conquer
- Approach: Break the problem into smaller subproblems, solve each subproblem recursively, and combine the solutions.
- Example: Merge Sort.
2. Dynamic Programming
- Approach: Solve complex problems by breaking them into simpler subproblems and storing their solutions to avoid redundant calculations.
- Example: Longest Common Subsequence.
3. Greedy Algorithms
- Approach: Make the best possible choice at each step, with the goal of finding the overall optimal solution.
- Example: Activity Selection Problem.
4. Backtracking
- Approach: Build solutions incrementally and backtrack when a solution cannot be completed.
- Example: N-Queens Problem.
Key Considerations:
1. Efficiency
- Time Complexity: Measures how the running time of an algorithm increases with the size of the input.
- Space Complexity: Measures how the memory usage of an algorithm increases with the size of the input.
2. Correctness
- Validity: An algorithm should correctly solve the problem for all valid inputs.
3. Simplicity
- Readability: Algorithms should be easy to understand and implement.
How Algorithm Works?
An algorithm works by following a specific sequence of steps to solve a problem or perform a task. Here’s a general overview of how algorithms function:
1. Define the Problem
Before designing an algorithm, clearly define the problem you want to solve. Understand the inputs, desired outputs, and constraints of the problem.
Also Read : What is neuroplasticity?
2. Design the Algorithm
Design the algorithm by outlining a step-by-step procedure to solve the problem. The design phase involves:
- Identifying Inputs and Outputs: Determine what data the algorithm will take in and what results it should produce.
- Breaking Down the Problem: Divide the problem into manageable parts or steps.
- Choosing the Approach: Decide on the algorithmic approach (e.g., sorting, searching, dynamic programming).
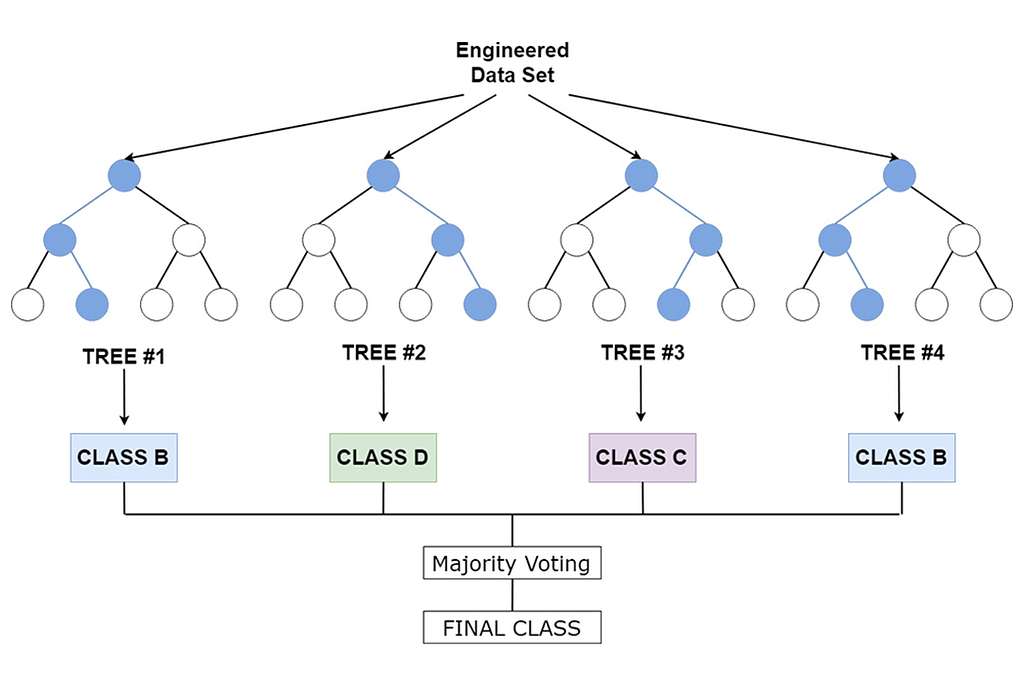
3. Write the Algorithm
Express the algorithm using pseudocode, flowcharts, or a programming language. The steps should be:
- Clear and Precise: Each step should be well-defined and unambiguous.
- Ordered: Steps must be executed in the correct sequence to achieve the desired result.
4. Implement the Algorithm
Translate the algorithm into code using a programming language. This involves:
- Writing Code: Implement the steps of the algorithm in a programming language.
- Debugging: Test the code to find and fix any errors.
5. Test the Algorithm
Run the algorithm with various test cases to ensure it works correctly:
- Correctness: Verify that the algorithm produces the correct output for all valid inputs.
- Edge Cases: Test with boundary or unusual cases to ensure robustness.
6. Analyze the Algorithm
Evaluate the algorithm based on:
- Time Complexity: How the execution time grows with the size of the input.
- Space Complexity: How the memory usage grows with the size of the input.
7. Optimize the Algorithm
If necessary, optimize the algorithm to improve efficiency:
- Optimize for Time: Reduce the time complexity if the algorithm is too slow.
- Optimize for Space: Reduce the space complexity if the algorithm uses too much memory.
Example: Sorting Algorithm (Bubble Sort)
Here’s how a simple sorting algorithm, Bubble Sort, works:
- Problem: Sort an array of numbers in ascending order.
- Design:
- Compare adjacent elements in the array.
- Swap them if they are in the wrong order.
- Repeat the process until the array is sorted.
- Algorithm (Pseudocode):
function bubbleSort(arr):
n = length of arr
for i from 0 to n-1:
for j from 0 to n-i-2:
if arr[j] > arr[j+1]:
swap(arr[j], arr[j+1])
- Implementation (Python):
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
- Testing:
- Test with arrays of different sizes and values.
- Verify that the array is sorted in ascending order.
- Analysis:
- Time Complexity: O(n^2) in the worst case, where n is the number of elements.
- Space Complexity: O(1) (in-place sorting).
- Optimization:
- Bubble Sort is not the most efficient sorting algorithm. Consider alternatives like Merge Sort or Quick Sort for larger datasets.
Conclusion
Algorithms work by providing a structured sequence of steps to solve problems. They are implemented in code and tested to ensure they perform as expected. Analyzing and optimizing algorithms is crucial to improve their efficiency and effectiveness. Understanding how algorithms work helps in designing better solutions and writing efficient code.
FAQ
1. What is time complexity?
Time complexity measures how the running time of an algorithm increases with the size of the input. It helps evaluate the efficiency of an algorithm.
2. What is space complexity?
Space complexity measures how the memory usage of an algorithm increases with the size of the input. It indicates the amount of memory required by the algorithm.
3. How do algorithms relate to data structures?
Algorithms often work with data structures to process and organize data. Choosing the right data structure can impact the efficiency and performance of an algorithm (e.g., using hash tables for quick lookups).
4. Can algorithms be implemented in different programming languages?
Yes, algorithms can be implemented in various programming languages. The choice of language may affect the implementation details, but the underlying algorithm remains the same.
5. Why is understanding algorithms important?
Understanding algorithms is crucial for designing efficient solutions, optimizing code, and solving complex problems effectively. It helps in making informed decisions about data processing, problem-solving strategies, and resource management.