Samsung ने हमेशा टेक्नोलॉजी की दुनिया में नए मानक स्थापित किए हैं, और उनका नया Samsung Galaxy Z Flip6 इस बात का एक और प्रमाण है। यह स्मार्टफोन न केवल अपने AI-संचालित फीचर्स के लिए जाना जाएगा, बल्कि इसकी कॉम्पैक्ट डिज़ाइन और शानदार प्रदर्शन इसे भीड़ से अलग बनाते हैं।
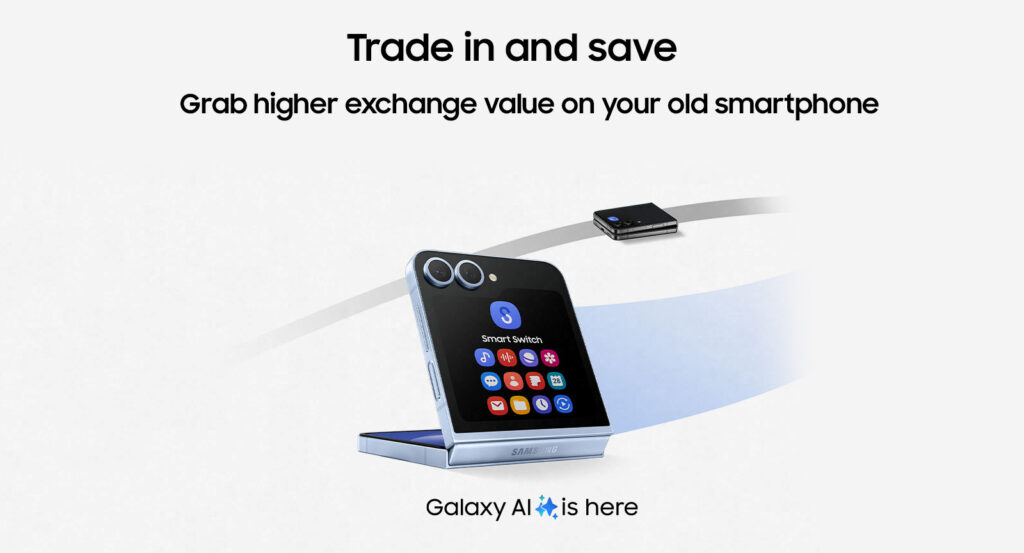
Main Specifications of Samsung Galaxy Z Flip6
- 12 GB RAM | 256 GB ROM
- 6.7 inch Full HD+ Display
- 50MP + 12MP रियर कैमरा और 10MP फ्रंट कैमरा
- 4000 mAh बैटरी
- Snapdragon 8 Gen 3 प्रोसेसर
Samsung Galaxy Z Flip6: Design and Performance
Samsung Galaxy Z Flip6 की डिज़ाइन न केवल स्टाइलिश है बल्कि compact भी है। इसका FlexWindow फीचर आपको फोल्डिंग डिस्प्ले का पूरा फायदा उठाने का मौका देता है। Snapdragon 8 Gen 3 प्रोसेसर के साथ यह स्मार्टफोन हाई-एंड गेमिंग और मल्टीटास्किंग के लिए परफेक्ट है।
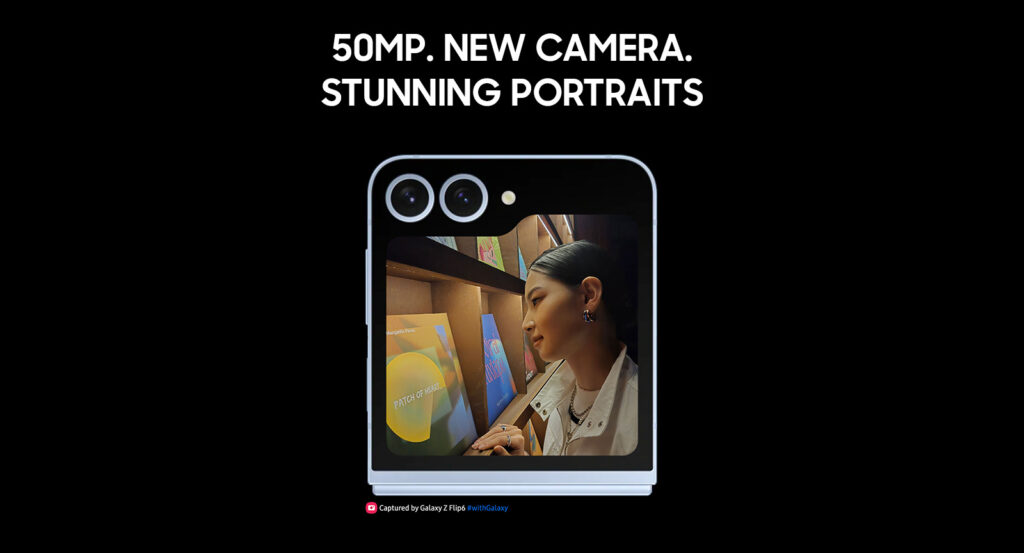
AI Powered Camera
Samsung Galaxy Z Flip6 स्मार्टफोन AI-बेस्ड कैमरा सिस्टम के साथ आता है। FlexCam फीचर आपके फोटोग्राफी अनुभव को और बेहतर बनाता है।
- Auto Zoom: Samsung Galaxy Z Flip6 का camera सब्जेक्ट पर फोकस रखते हुए camera खुद ही जूम इन या आउट कर लेता है।
- Hands-Free Selfie: FlexWindow में प्रीव्यू के साथ बिना हाथ लगाए फोटो खींचें।
इसके अलावा, Galaxy AI आपके हर शॉट को परफेक्शन तक ले जाने में मदद करता है।
Long Lasting Battery Life
Samsung Galaxy Z Flip6 में 4000 mAh की बैटरी दी गई है, जो इसे Flip सीरीज का सबसे लंबा बैटरी बैकअप देने वाला स्मार्टफोन बनाती है। इसके साथ Snapdragon 8 Gen 3 प्रोसेसर बैटरी की efficiency को और बढ़ाता है, जिससे आप दिनभर गेमिंग, कॉलिंग या टेक्स्टिंग कर सकते हैं।
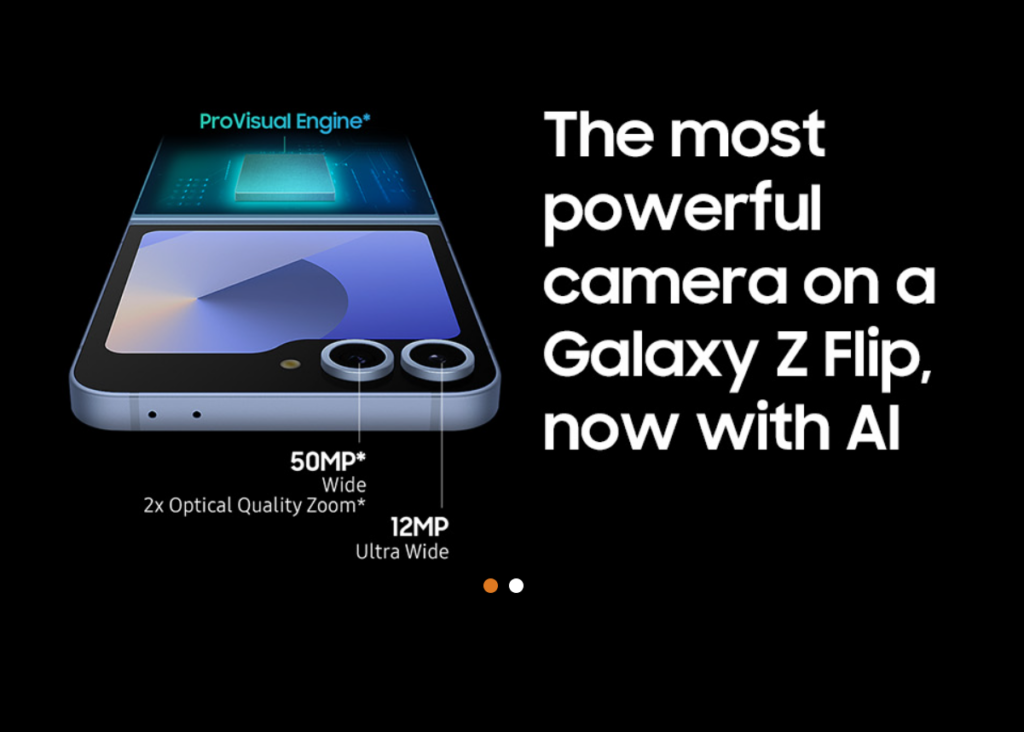
Durability of Galaxy Z Flip6
स्मार्टफोन में IP48 वाटर रेजिस्टेंस है, जो इसे पानी के छींटों से सुरक्षित रखता है। इसके अलावा, Corning Gorilla Glass Victus 2 स्क्रीन को खरोंच और टूट-फूट से बचाता है।
Inside the Box
स्मार्टफोन के साथ दिए जाने वाले आइटम्स:
- Handset
- डेटा केबल (C to C)
- सिम इजेक्शन पिन
- Quick स्टार्ट गाइड
- प्रोटेक्शन फिल्म (केवल मेन स्क्रीन के लिए)
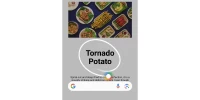
Also check: Samsung Galaxy S23 FE: Moments Made easy to capture
Specification Table
Feature | Details |
---|---|
Model Name | Galaxy Z Flip6 5G |
Processor | Snapdragon 8 Gen 3 |
Operating System | Android 14 |
Battery | 4000 mAh |
Display | 6.7-inch Full HD+ |
Main Camera | 50MP + 12MP |
Front Camera | 10MP |
Water Resistance | IP48 |
Glass Protection | Corning Gorilla Glass Victus 2 |
AI Feature with Samsung Galaxy Z Flip6
Galaxy AI आपकी टेक्स्टिंग और फोटोग्राफी को एक नया पहलू देता है।
- Chat Assist: FlexWindow से सीधे मेसेज भेजें और AI के सजेस्टेड रिप्लाई का उपयोग करें।
- Self-Expression Tool: AI और 50MP प्रो-लेवल कैमरा के साथ अपनी क्रिएटिविटी को बढ़ावा दें।
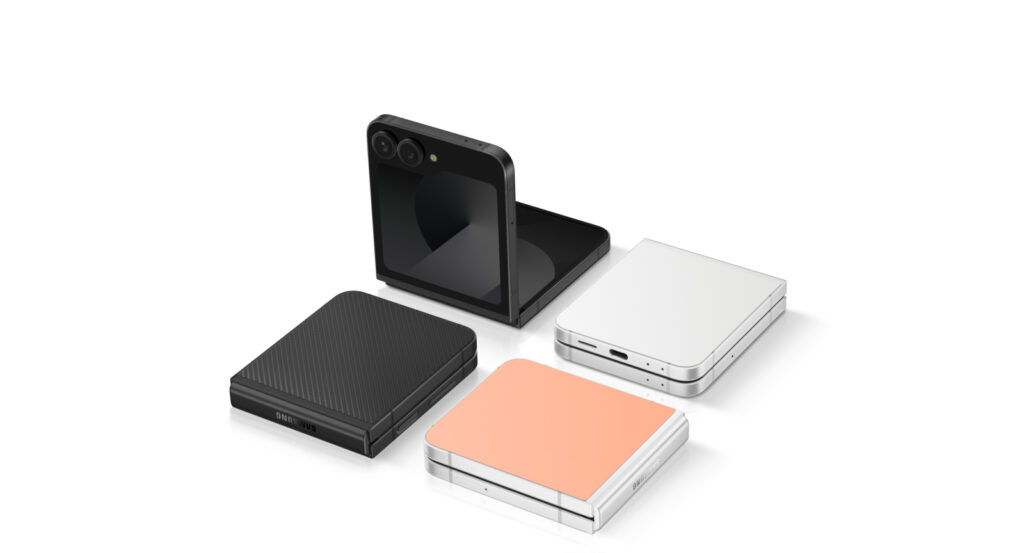
Conclusion
Samsung Galaxy Z Flip6 उन लोगों के लिए है, जो स्टाइल, परफॉर्मेंस और इनोवेशन को साथ लेकर चलना चाहते हैं। इसके AI-समर्थित फीचर्स और टिकाऊ डिज़ाइन इसे स्मार्टफोन मार्केट का असली गेम-चेंजर बनाते हैं।